Learning Game Development: Complete guide using HTML5, CSS & JavaScript
September 22nd, 2021 10:54 AM Mr. Q Categories: JavaScript, Learning
Game development steps start to finish; layout of everything needed to create JavaScript games. Using the three pillars of Object Oriented Programming, (Encapsulation, Inheritance, & Polymorphism) to keep our code clean and easy to maintain. Why use JavaScript for game programming: #1 answer is, it will run on any device that supports a modern browser. Source control is in scope for this article, but not the focus. Supporting links will be included for the supporting game posts,
Click the links below to learn how to install the required tools: (at the moment, I only have a Windows box. If you have a Mac or Unix, would you be nice enough to share the steps?)
This is a work in progress (WIP), this comment will be removed when complete.
Integrated Development Environment (IDE)
An IDE is used to help develop your code. A feature that you should consider is IntelliSense which will help with your code completion.
- Visual Studio Code
- (This is what we will be using for our development)
- Install video with Web Server
- Eclipse
- IntelliJ
- NetBeans
- Visual Studio
Required JavaScript commands, HTML5 and CSS to learn
This will not be a JavaScript in-depth. We will focus only on the commands needed to write games. In addition, we will use a JavaScript, HTML5, and minimal CSS.
HTML
- DOM
CSS
- border
- margin
- padding
JavaScript
- alert
- Console.______
- let
- Understanding Scope of Visibility in JavaScript Programming
- for
- deltaTime
- Array
- push
- pop
- Class (Object Oriented Programming OOP) in JavaScript
- Understanding Classes in JavaScript: Functions, Methods, Access Modifiers, and Lifecycle
- The power of classes in javascript (Encapsulation, Inheritance, & Polymorphism)
- window.addEventListener
- Canvas object – Context for 2D
- clearRect
- fillRect
- width
- height
- font
- fillStyle
- textAlign
- fill
- fillText
- requestAnimationFrame
- beginPath
- closePath
- lineWidth
- stroke
- arc
- strokeStyle
- globalAlpha
Game Engine Loop
As needed, additional capabilities will be added to the game engine loop. An example of capabilities to add are sprites, input controls (keyboard & joystick), collision detections, sound, tilemap, pan/scroll, parallax scrolling background, and Artificial Intelligence. These will all be turned into reusable classes or a framework to use.
Game States:
- attract
- playerSelect
- initGame
- initEnemy
- playGame
- pauseGame
- gameOver
Methods:
- Input
- keyboard
- mouse
- GamePad
- Update
- Draw
Game Engine Math
Supporting classes will be needed, these topics will be covered as they are needed and then extended to add capabilities.
- Math
- Random
- PI
- SQRT
- sin
- cos
- Physics
- Gravity
- Collision detections
- Pathfinding
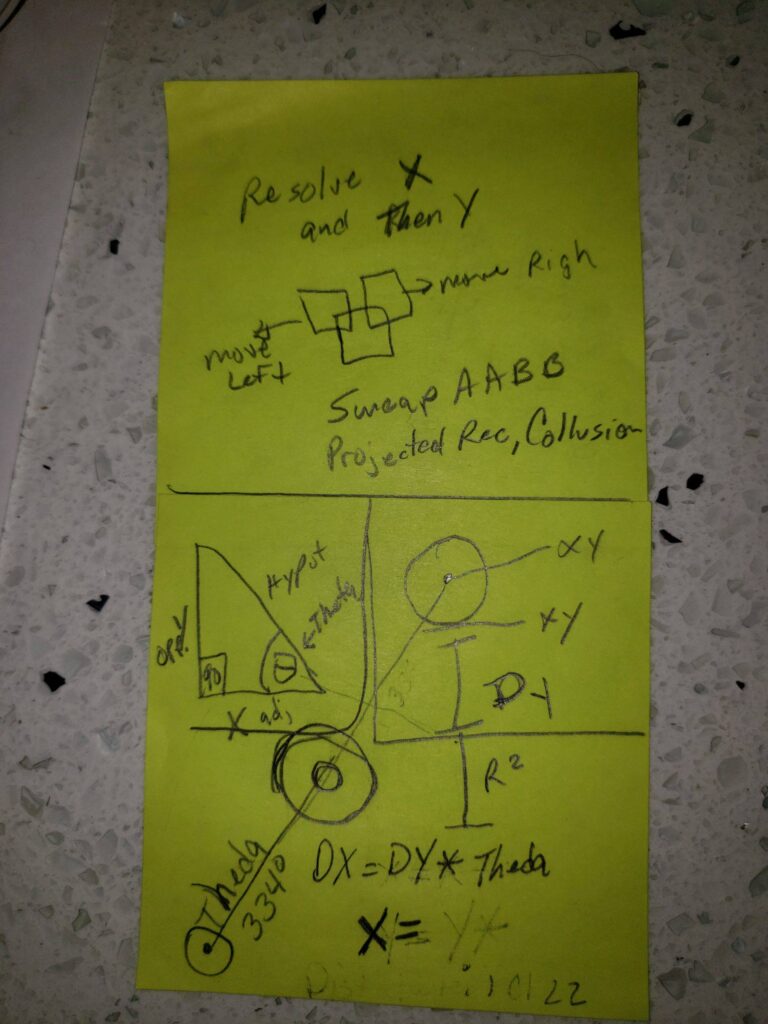
Games to develop
Where available, the original artwork will be convertied to sprites (animated bitmap frames).
Tic-Tac-Toe
- Complexity: Very Low
- Reason: Straightforward game loop and simple win condition checks with a 3×3 grid.
- Complexity: Very Low
- Reason: Requires basic 2D array manipulation and simple state transitions based on fixed rules.
Connect 4
- Complexity: Low
- Reason: Slightly more complex grid-based logic than Tic Tac Toe, requiring checks for vertical, horizontal, and diagonal matches.
- Complexity: Low
- Reason: Introduces basic collision detection, ball physics, and simple AI for paddle movement.
Snake
- Complexity: Medium
- Reason: Requires handling a dynamic array for the snake’s body, collision detection with self and boundaries, and input handling.
- Complexity: Medium
- Reason: Involves circular motion (using trigonometry), managing multiple moving objects, and possible scaling issues for visualization.
Space Invaders
- Complexity: Medium
- Reason: Involves handling multiple enemies, shooting mechanics, and basic enemy AI with predictable patterns.
Asteroids
- Complexity: Medium
- Reason: Requires handling physics for rotation and thrust, collision detection with irregular shapes (asteroids), and screen wrapping.
Tanks
- Complexity: Medium to High
- Reason: Involves player and enemy movement, shooting mechanics, and terrain handling with simple line-of-sight AI.
Frogger
- Complexity: Medium to High
- Reason: Managing multiple lanes of traffic and logs with different speeds, collision detection, and timing elements.
Tetris
- Complexity: High
- Reason: Complex grid manipulation with collision checks, shape rotation, line clearing, and increasing speed.
Dig Dug
- Complexity: High
- Reason: Involves complex digging mechanics, enemy AI, and interactions between player, enemies, and the environment.
Donkey Kong
- Complexity: High
- Reason: Requires platform mechanics, barrel behavior, jump physics, and interaction between player and various obstacles.
Mario
- Complexity: Very High
- Reason: Involves intricate platforming physics, collision detection, advanced enemy AI, power-up management, level design, and animation.
Pac-Man
- Code Complexity:
- Reason:
- Pathfinding AI (High): Requires implementing algorithms like A* or BFS for ghost behaviors, with distinct strategies for each ghost (e.g., chase, ambush, random movement).
- Collision and State Management (High): Handles collision detection for walls, power pellets, and interactions between Pac-Man and ghosts, including state changes (normal, frightened, eaten).
King of the Ice Berg (an original game)
- Complexity: Very High
- Reason: Likely features unique game mechanics, complex interactions, and possibly non-standard physics or AI, making it challenging to implement.
Application Server
For your games to work, you need to host them on an application server. Any application server will work. The files that we created need to be stored in the root directory/folder. Below is a short list which you can choose from.
- Apache
- Bitnami with WordPress
- IIS Express (included with Visual Studio)
- XAMPP
Reference
- HTML5 – Canvas
- MDN Web Docs
- MDN Games
- W3Schools JavaScript
- https://www.youtube.com/watch?v=5E5bzjcKx5w
Visual Studio 19 – C:\Users\{user}\source\repos